]
---
## 2. Interactive plotting with .red[*bokeh*], a Python package
### Metal-organic frameworks as drug encapsulators
.col31[

]
--
.col32[
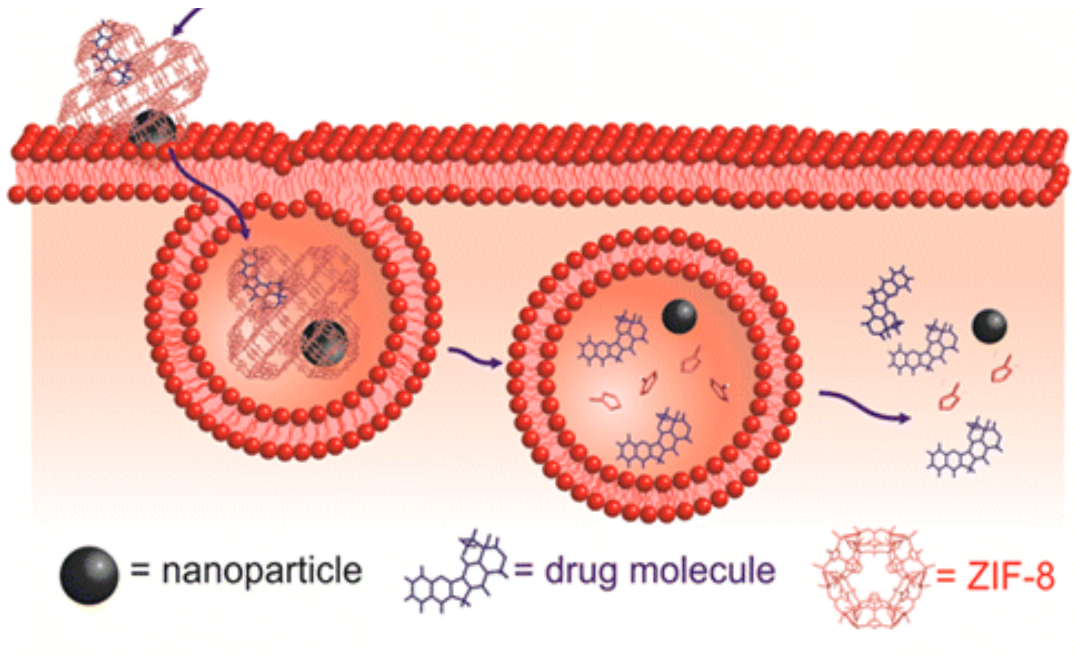
]
--
.col33[
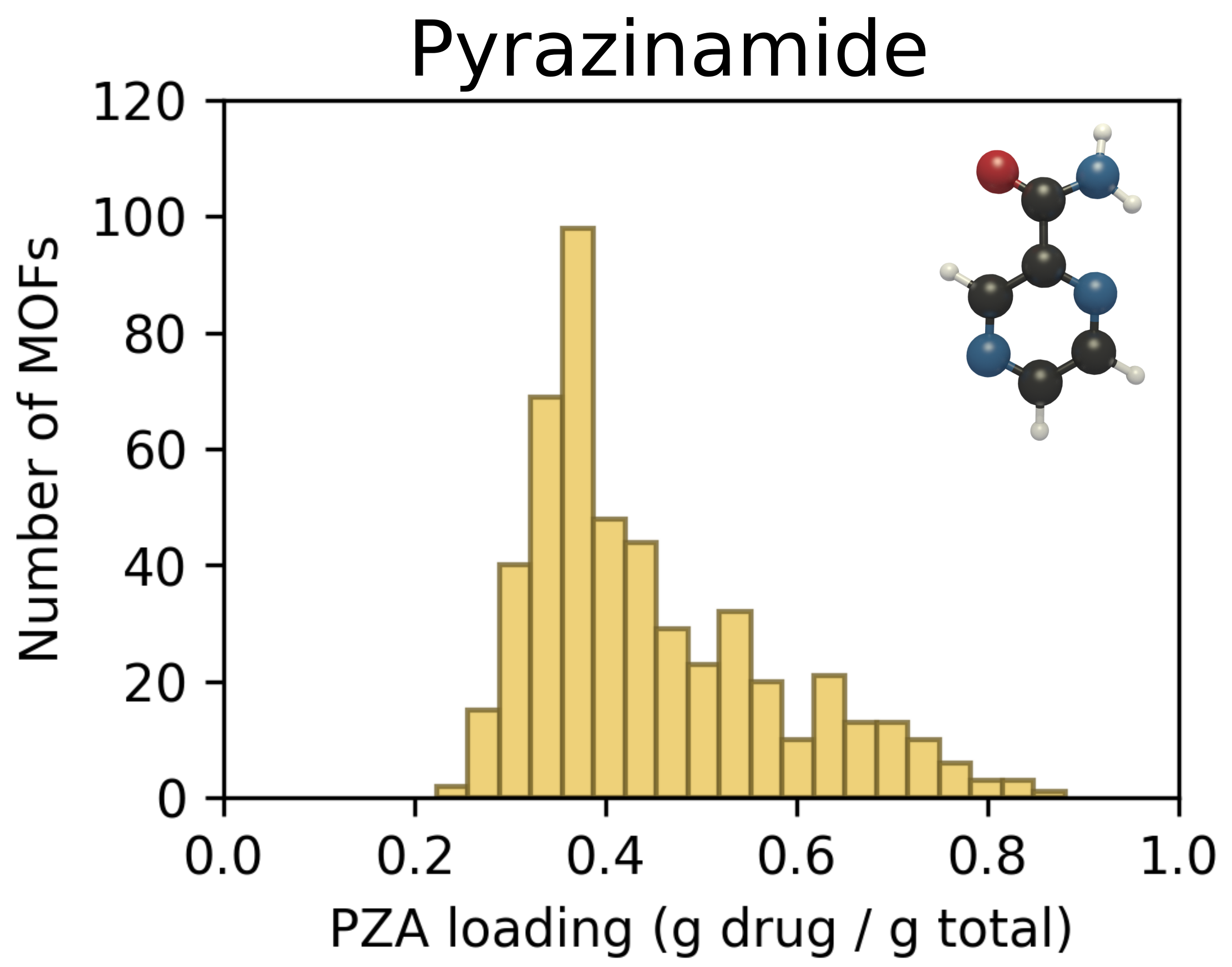
]
Pyrazinamide is a medication used to treat tuberculosis
--
### Objective
- Collaborators want to see which MOF they can synthesize
- Make an interactive plot to show PZA uptake and properties of each MOF
---
### .red[Start Jupyter Notebook]
- Windows: Start Anaconda prompt
- Mac/Linux: Start terminal
```python
jupyter notebook
```
--
### .red[Read data (csv)]
#### .gray[Make sure to copy downloaded data folder to current directory]
```python
import os, csv
rows = []
csvfile = os.path.join('data', 'pza-uptake.csv')
with open(csvfile) as fileobj:
reader = csv.reader(fileobj)
for row in reader:
rows.append(row)
```
---
### .red[Arrange data]
```python
# Headers in the same order as the csv file
headers = ['mof', 'metal', 'pza', 'err', 'vp', 'vf', 'ro', 'sa', 'dia']
# Create a dictionary where each header is assigned to all column values
# This syntax uses dictionary and list comprehensions
data = {h: [i[idx] for i in rows[1:]] for idx, h in enumerate(headers)}
# Convert numerical values to float (csvreader reads as string by default)
keys = ['pza', 'err', 'vp', 'vf', 'ro', 'sa', 'dia']
for k in keys:
data[k] = [float(i) for i in data[k]]
```
--
### .red[Plot data]
```python
from bokeh.plotting import figure, show
from bokeh.plotting import output_notebook
p = figure()
p.circle(data['vp'], data['pza'])
show(p)
output_notebook()
```
---
### .red[Customize figure]
#### .gray[Labels and size]
```python
p = figure(plot_width=1000, plot_height=600,
title='Pyrazinamide Uptake (g PZA/ g MOF)')
p.xaxis.axis_label = 'Pore Volume (cm³/g)'
p.yaxis.axis_label = 'Pyrazinamide uptake (g PZA/ g MOF)'
```
--
#### .gray[Markers]
```python
p.circle(data['vp'], data['pza'],
size=18,
fill_color='salmon',
fill_alpha=0.5,
line_color="darkred")
```
-
See available colors here
---
### .red[Hover tool]
#### .gray[Collect image files]
```python
import os
# For viewing the plot locally on your computer
img_dir = os.path.join('data', 'mofs')
data['images'] = [os.path.join(img_dir, '%s.svg' % i) for i in data['mof']]
# For viewing plot in the webpage
img_dir = 'assets/img/mofs'
data['images'] = ['%s/%s.svg' % (img_dir, i) for i in data['mof']]
```
---
### .red[Hover tool]
#### .gray[Create hover tool]
```python
from bokeh.models import HoverTool
hover = HoverTool(
tooltips="""
@mof
"""
)
```
---
#### .gray[Customize toolbar]
```python
p = figure(plot_width=1000, plot_height=600,
title='Pyrazinamide Uptake (g PZA/ g MOF)',
tools=[hover, "pan", "wheel_zoom", "box_zoom", "reset", "tap"],
toolbar_location='right')
```
--
#### .gray[Add data source, hover tool and plot]
```python
from bokeh.plotting import ColumnDataSource
source = ColumnDataSource(data=data)
p.circle('vp', 'pza',
source=source,
size=18,
fill_color='salmon',
fill_alpha=0.5,
line_color="darkred")
# We need to save to html file to make the images visible
output_file('pza.html')
```
---
#### .gray[Add more info to hover html]
```python
hover = HoverTool(
tooltips="""
@mof
Metal:
@metal
Vf:
@vf
Surface Area:
@sa
Pore diameter:
@dia
"""
)
```
---
#### .gray[Highlight selection]
```python
p.circle('vp', 'pza',
source=source,
size=18,
fill_color='salmon',
fill_alpha=0.5,
line_color="darkred",
nonselection_fill_color="salmon",
nonselection_line_color="darkred",
nonselection_fill_alpha=0.5,
nonselection_line_alpha=1.0,
selection_fill_alpha=0.5,
selection_fill_color="blue",
selection_line_color="darkred")
p.ygrid.grid_line_alpha = 0.2
p.xgrid.grid_line_color = None
# We need to save to html file to make the images visible
output_file('pza.html')
```
--
#### .red[Embed html]
```python
from bokeh.resources import CDN
from bokeh.embed import file_html
html = file_html(p, CDN, "PZA uptake")
with open('pza.html', 'w') as html_file:
html_file.write(html)
```
---
class: center, middle
# .center[
Amazing!
]
---
## 3. Adding the plot to the webpage
- Go to your GitHub page repository.
- Add the html file using `Upload files` button.
- Add the MOF images to `assets/img` folder again using `Upload files` button.
- Edit `_config.yml` file and add your file to the navigation bar. The url should be the same as your html file name. Ex: url for `plot.html` should be `plot`.
- The plot should be available by clicking the link in the sidebar or through `
.github.io/`.
#### .red[It should look something like this: [click here](pza-uptake)]
---
## 4. Adding an interactive molecule to the webpage
- Go to molview: [http://molview.org/](http://molview.org/)
.center[
]
- Draw any molecule you like or just use the default caffeine
- If you draw your own molecule click on `2D to 3D` after you are done
- Click `Tools -> Embed`
- Copy the HTML code
- Paste the code to any markdown or html file
---
class: center, middle
# .center[Caffeine
]
More information on adding interactive molecules: [click here](https://kbsezginel.github.io/research/web-molecules/)
---
class: center, middle
#